Before or after you start with this sample please first take a look on this MSDN article. This example describes the idea of application which is running in the background.
Unfortunately this all will not work if follow steps described in article or it will work a bit differently.
First of all, background processing is designed for Location aware applications. That means by default you cannot use this technical design on Windows Phone to implement some other kind of application which is running in the background.
I have experimented with this behavior and found the how to implement background processed even if no location is in play.
let’s build an application which will continue running after you navigate away from your application. To do that go to your MainForm.cs (or any other) and add following code:
void MainPage_Loaded(object sender, RoutedEventArgs e) { new Thread (delegate() { while (true) { Debug.WriteLine(DateTime.Now.ToString()); Thread.Sleep(2000);// Do some Job. } }).Start(); } |
This code solely simulate some background processing.
Then open WMAppManifest.xml in XML editor and append following snippet:
<Tasks>
<DefaultTask Name ="_default" NavigationPage="MainPage.xaml">
<BackgroundExecution>
<ExecutionType Name="LocationTracking" />
</BackgroundExecution>
</DefaultTask>
</Tasks>
Note that the name “LocationTracking” is mandatory! If you enter something else it will not work. LocationTracking is system predefined execution type and not a name which can be freely chosen.
When you start the application in debugger the output window will show something like:
This indicates that your background job is running while application is running. By design of Background-Processing this thread should continue running when you navigate away from your application. For example click “Windows Key” (Home Key) or seaerch or let somebody call you. If you do that you will figure out that your application will be suspended. This is common behavior which will apply to all applications anyhow. The BackgroundExecution tag notifies the system that navigating out of application should keep the application running and not to suspend it. In this case (which does not work) you will see following output:
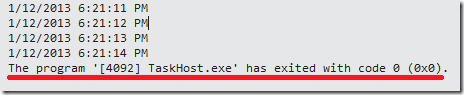
This means that application has been suspended. And this is exactly what you don’t want for sure. In fact you did all right. The problem is that Microsoft has designed this background worker behavior for Location Services only! Yes this is TRUE!
That means you cannot by design implemented some thread which is doing some job.
To approve this I just removed my thread and added the code which is tracking the location. Manifest remains as it is.
Geolocator geolocator; void MainPage_Loaded(object sender, RoutedEventArgs e) { geolocator = new Geolocator(); geolocator.DesiredAccuracy = PositionAccuracy.High; geolocator.MovementThreshold = 100; geolocator.PositionChanged += geolocator_PositionChanged; } void geolocator_PositionChanged(Geolocator sender, PositionChangedEventArgs args) { Debug.WriteLine("location changed.."); } |
Start the application, open location emulator and click somewhere in the area which is larger than movement threshold. Just chose bigger distances between points on map.
Now press Search-Key and Look what happened now:
The process which is hosting your application no more unloaded and location tracking is still working while you are changing position on the map.
This is good news, but unfortunately you don’t want to write Geo-Application. :(
No problem, just do following now:
1. Keep the Geolocation related code. Just change MovementThreshold to some really big value like 1000000 and set accuracy to Default.
By such settings you will practically startup GPS sensor, but you will put it into sleep mode.
2. Get back the Thread code which implements your job.
The code at the end should look like:
Geolocator geolocator; void MainPage_Loaded(object sender, RoutedEventArgs e) { new Thread (delegate() { while (true) { Debug.WriteLine(DateTime.Now.ToString()); Thread.Sleep(2000);// Do some Job. } }).Start(); geolocator = new Geolocator(); geolocator.DesiredAccuracy = PositionAccuracy.Default; geolocator.MovementThreshold = 100000; geolocator.PositionChanged += geolocator_PositionChanged; } void geolocator_PositionChanged(Geolocator sender, PositionChangedEventArgs args) { Debug.WriteLine("location changed.."); } |
Now start the application, press “Search-Key” and look in output window:
One more thing at the end.
If you like you can define the handler which will notify you that you are running in the background (snippet from App.xaml)
<Application.ApplicationLifetimeObjects>
<!--Required object that handles lifetime events for the application-->
<shell:PhoneApplicationService
Launching="Application_Launching" Closing="Application_Closing"
Activated="Application_Activated" Deactivated="Application_Deactivated"
RunningInBackground="Application_RunningInBackground"
/>
</Application.ApplicationLifetimeObjects>
Unfortunately this handler is not always invoked. In some apps works fine, but in most apps is mostly not called. So, I don’t use it.
Fortunately you can use Application_Deactivated.
// Code to execute when the application is deactivated (or sent to background)
// This code will not execute when the application is closing
private void Application_Deactivated(object sender, DeactivatedEventArgs e)
Posted
Jan 15 2013, 10:41 AM
by
Damir Dobric