To send telemetry data from device, we will use Microsoft.Azure.Devices.Client or generally one of following two packages:
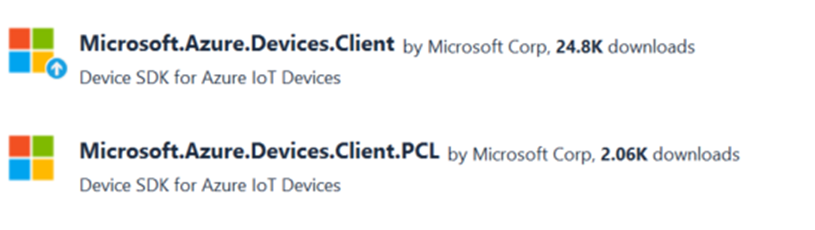
First one is used when programming devices on a full desktop (typically simulators). If your device is running on UWP you will use the PCL package.
Before we send telemetry data to IotHub (or event hub), we need to define how the data will look like. In this example, we will simulate data obtainer from 3 sensors, which can be described as following anonymous object:
var telemetryData = new
{
deviceId = "D001",
Temperature = temperature,
Current = strom,
Location = geoData[indx],
};
It is a message, which contains temperature, current and position of device. It basically simulates data obtained from 3 sensors.
As next, the message has to be converted to string by using of following line:
var messageString = JsonConvert.SerializeObject(telemetryDataPoint);
Note, that we have used JSON format in this case, which is commonly widely used. If required, you can use any other format like XML or binary. However, using of JSON is recommended, because most cloud tools (i.e.: Azure Stream Analytics) provide JSON support rather than XML. In any case, you can use the format of your choice. Azure IoTHub does not require you to use any specific format.
However, the message cannot be created from string directly. Every message sent to IoTHub must be converted in byte array, from which the actual message will be created. With that approach message creator is responsible for definition of the contract, encoding and format of the message.
var message = new Message(Encoding.UTF8.GetBytes(messageString));
Please note that when using IotHub api (as in this example), you cannot specify partition key. Partition key is under the hub a part of the message, which you cannot se here. It is a string value, which is used as a hash-key to assign the message to specific partition. When using IotHub all messages send from a single device are automatically hashed with the same partition key. This leads to sending of messages from a single device to a single partition.
If you want to change this, you can use ServiceBus client SDK. But this is out of scope of this post.
Once we have a message serialized and encoded as byte array method SendAsync can be used to send it. Additionally to SendAsync, method SendBatchAsync can be sued to send a batch of messages.
await m_DeviceClient.SendEventAsync(message);
Following picture shows the message serialized as JSON.
![clip_image002[4] clip_image002[4]](http://developers.de/cfs-file.ashx/__key/CommunityServer.Blogs.Components.WeblogFiles/damir_5F00_dobric/clip_5F00_image0024_5F00_thumb_5F00_4548A3E9.jpg)
Method below shows complete implementation of sending of simulated telemetry messages to IotHub.
Random rand = new Random(); List<string> geoData = new List<string>(); geoData.Add("Frankfurt am Main");// 50.1109901,8.6828398"); geoData.Add("Seattle");// 47.6147628,-122.475987"); while (true) { double strom = rand.Next(1, 5) + rand.NextDouble(); double temperature = rand.Next(30, 70); var indx = rand.Next(0, 2); var telemetryDataPoint = new { deviceId = "D001", Temperature = temperature, Current = strom, Location = geoData[indx], }; var messageString = JsonConvert.SerializeObject(telemetryDataPoint); var message = new Message(Encoding.UTF8.GetBytes(messageString)); await m_DeviceClient.SendEventAsync(message); Console.WriteLine($"{DateTime.Now} > Sending message: {messageString}"); Thread.Sleep(5000); }
|
Posted
May 25 2016, 06:07 AM
by
Damir Dobric