ResourceManagementClient is a class inside of Microsoft.Azure.Management.ResourceManager, which provide simplified API over Azure Resource Manager.
In this post I will show a short example, which demonstrates how to instantiate this class. First of, we have to obtain authorization token. There are several ways to do that. Next code sample shows the hard (native) way to obtain a token from Service Principal credentials. That means, the following code should be executed from some backend service.
public static async Task<string> GetTokenFromAadWithSecretKeyAsync(string secret, string resourceUrl, string clientId, string tenantId, AzureEnvironment environment = null) { if (environment == null) environment = AzureEnvironment.AzureGlobalCloud; var client = HttpFactory.GetHttpClient(environment.AuthenticationEndpoint); string tokenEndpoint = $"{tenantId}/oauth2/token"; var body = $"resource={resourceUrl}&client_id={clientId}&grant_type=client_credentials&client_secret={secret}"; var stringContent = new StringContent(body, System.Text.Encoding.UTF8, "application/x-www-form-urlencoded"); var response = await client.PostAsync(tokenEndpoint, stringContent); if (response.StatusCode == HttpStatusCode.OK) { JObject jobject = JObject.Parse(response.Content.ReadAsStringAsync().Result); var token = jobject["access_token"].Value<string>(); return token; } else throw new Exception(response.Content.ReadAsStringAsync().Result); }
|
Credentials are defined by the secret and clientId. The client Id is also known as ApplicationId and it can be copied from AAD blade of your application.
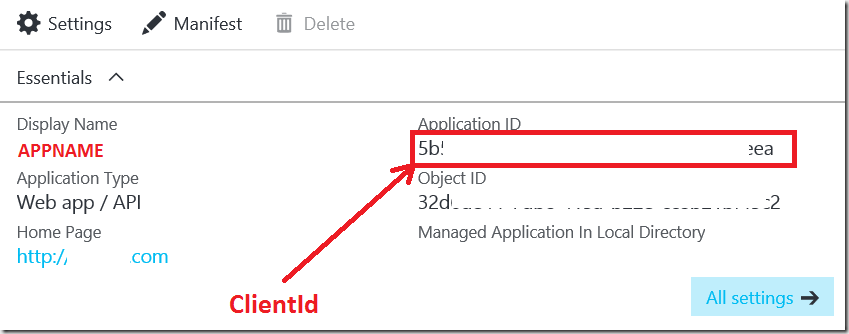
Secret can be obtained from the same blade:
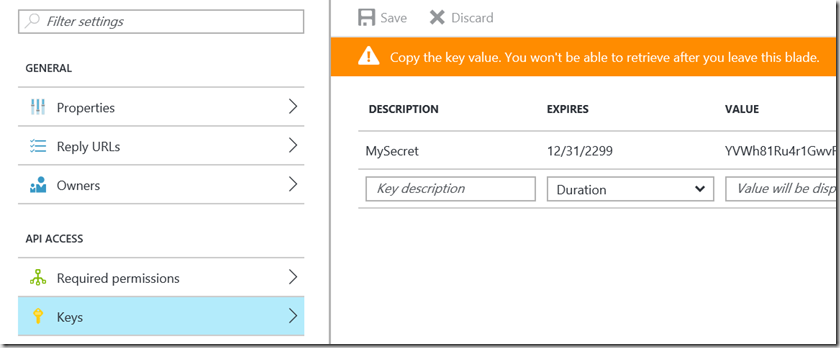
Assuming that you have tenantId, you will have to specify correct resourceUrl. Resource URL defines the service URL, which you want to access with the token.
In a case of Azure Resource Manager this URL is defined by AzureEnvironment.ManagementEnpoint. ManagementEndpoint property of AzureEnvironment is in Powershell called ServiceManagementUrl.
In a case of public cloud this is https://management.core.windows.net/. In a case of German Cloud this is https://management.core.cloudapi.de. As you see it is different URI in different environments.
Another, easier option to create credentials is:
var serviceCreds = await ApplicationTokenProvider.LoginSilentAsync(tenantId, clientId, secret); |
One you have a token, you can start coding. Following snippet shows instantiation.
public async void Run(string deploymentName, string token, Uri authEndpoint, string subscriptionId, string resourceGroupName, string resGroupLocation, string jsonTemplate, string jsonParams) { // Try to obtain the service credentials var serviceCreds = new TokenCredentials(token); // Read the template and parameter file contents JObject templateFileContents = JObject.Parse(jsonTemplate); JObject parameterFileContents = JObject.Parse(jsonParams); // Create the resource manager client var resourceManagementClient = new ResourceManagementClient(serviceCreds); resourceManagementClient.SubscriptionId = subscriptionId; // Create or check that resource group exists ensureResourceGroupExists(resourceManagementClient, resourceGroupName, resGroupLocation); // Start a deployment deployTemplate(resourceManagementClient, resourceGroupName, deploymentName, templateFileContents, parameterFileContents); }
|
Posted
Mar 28 2017, 07:16 AM
by
Damir Dobric