No question IoT (Internet of Things) seems to be very interesting, usable and powerful topic. When talking about IoT we typically think about small devices and cool solutions around them in human accessible environment.
Interestingly, there is an initiative in Germany called “Industrie 4.0”, which massively expand the definition of usage of “things". According to this initiative information collected from small devices should have an impact to almost every sphere of humans life (meaning positive). That means sensors big data needs to be collected and processed in human usable information in social and collaboration context.
This definition bring us to a large number of new high-level scenarios, which can be implemented by Share Point developers.
By following this definition I would like to set the general simplified model of IoT in context of Share Point.
The picture shown below, defines the problem domain in which we will focus in this article. We typically have a device, which implements lover-level functionality. The data produced by device needs to be sent to SharePoint and Some Commands (or data) needs to be sent from SharePoint to device.
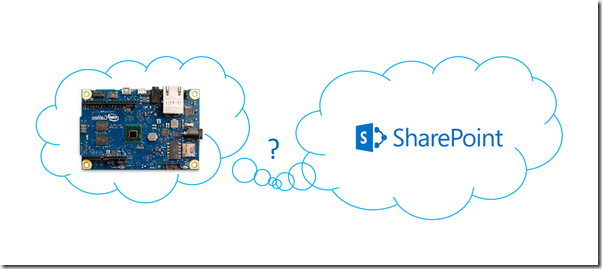
According to defined scope of this scenario we can start to elaborate many issues, which needs to be fixed to make this working. In my concrete scenario I want to use a Gas Sensor which will autonomously publish gas measure values to SharePoint. Additionally I want be able to take a control over Gas Sensor directly from SharePoint.
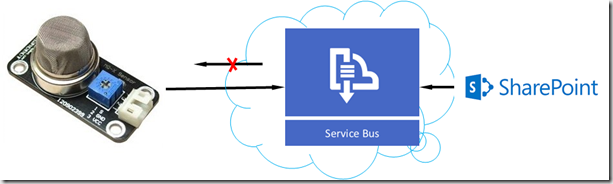
To solve the problem I will use Service Assisted Communication with Azure Service Bus, which Clemens briefly described in this post. Here are few facts related to this kind of connectivity:
-In this communication sensor will not host the web server or any other server.
- Because no server is used, we can use chipper devices. (server is a big thing)
- If there is no server on the sensor side we do not need an IP address.
No IP Address no problems with IP4 and IP6 address range.
- If the sensor is not accessible on the network (it is not a server) then it is more secured.
- All traffic will be encrypted by SSL.
- As authentication/Authorization token the Service Bus SAS will be used.
- I will use SharePoint online and an SharePoint app, which will be implemented as HTML5/Java Script.
However this app will act as service (not a SharePoint ) itself. It means the HTML code will star receiving of
sensor data from Service Bus.
- I will use another HTML5/JavaScript SharePoint App to send commands to the sensor via Service Bus.
- To implement bindings etc. in the app front I will use AngularJS.
- For communication with the service bus from JavaScript I will use Service Bus Java Script SDK.
In following few topics I will explain how to build the Gas Sensor Module (only software part) and how to implement SharePoint App which bidirectional communicate with Gas Sensor.
Gas Sensor
The sensor is implemented as module in .NET Micro framework. For this purpose I used the project template
.NET Gadgeteer Module
To be able to make this in Visual Studio I installed following GHI software with gadgeteer packages and .NET Micro framework
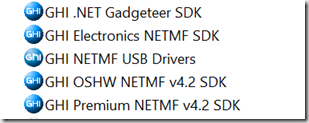
Because I’m going to implement the module independent on Micro framework version I added a GasModule.cs file and linked it to every module version. Linked file is marked with red frame. All other files are automatically created by project template.
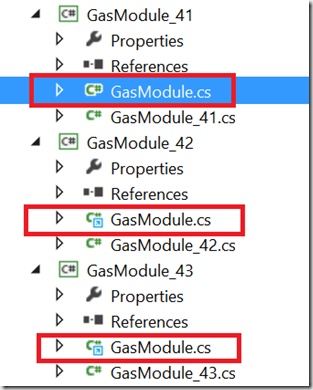
The GasSensor module provides few public method in very simple API. Sensor can be similarly to GPS API read by calling GetVoltage() method. This method returns the voltage from 0-3,5 Volt. The higher gas intensity measured, the higher voltage.
Additionally you can start and stop of reading gas values by calling Start() and Stop() respectively. We can also set the threshold value (in percent) which defines the threshold voltage. When threshold voltage is reached and Start() previously has been called the sensor will automatically fire event via OnThresholdReached.
Implementation hardware details are out of scope of this post.
Then I will create a new Gadgeteer application with FEZ Spider Board (You can also use any other gadgeteer compatible hardware).
Then I will add in gadgeteer designer my GasSensor module and connect it to the board.
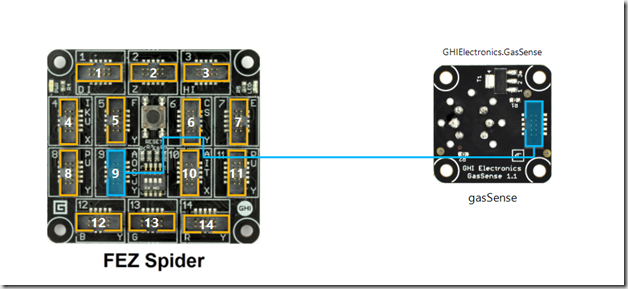
Following code shows the full implementation of the application which uses GasModule describe above.
The code is spitted in two parts:
1. Sending of measured voltage if threshold is reached.
2. Receiving of commands (stop, start and threshold)
When program is started we will subscribe sensor events which will be fired when the gas reaches default value, which is 80% of maximum voltage of 3,5V. As next, we will call start() to start continuous reading process in sensor module itself. Every time the event is fired we will send the data (ingress) to the Service Bus queue. See method publishCriticalEvent().
For sending of events I will use MessagingClient, which I will reference later. This class is very similar to QueueClient and TopicClient in .NET SB SDK.
Then we create a new thread which runs in method startReceiveCommandLoop. The code provided below shows the full bidirectional communication with (in this case) SharePoint via Service Bus broker.
public partial class Program { private GasModule m_Gas; // This method is run when the mainboard is powered up or reset. void ProgramStarted() { m_Gas = new GasModule(1, 2); m_Gas.OnThresholdReached += gas_OnThresholdReached; m_Gas.Start(); new Thread(() => { startReceiveCommandLoop(); }).Start(); // Use Debug.Print to show messages in Visual Studio's "Output" // window during debugging. Debug.Print("Program Started"); } /// <summary> /// Invoked when gas has reached defined threashold value. /// </summary> /// <param name="sender"></param> /// <param name="state"></param> void gas_OnThresholdReached(GasModule sender, ThresholdReachedEventArgs state) { publishCriticalEvent(state); } /// <summary> /// Sending sensor state. /// </summary> /// <param name="state"></param> private static void publishCriticalEvent(ThresholdReachedEventArgs state) { var settings = new ApplicationSettings("gasingressqueue"); var tokenProvider = new TokenProvider(settings.DeviceAccount, settings.DeviceKey); var sendClient = new MessagingClient(settings.EventSubmissionUri, tokenProvider); try { var sensorMsg = new SimpleMessage { Properties = { { "gaslevel", state.Threshold}, {"timestamp", state.Timestamp}, {"sensorid", state.SensorId}, {"owner", "Damir Dobric – daenet GmbH"}} }; sendClient.Send(sensorMsg); Debug.Print("New state sent: " + state.Timestamp.ToString() + ", " + state.Threshold); } catch (Exception ex) { } } /// <summary> /// Receiving commands. /// </summary> private void startReceiveCommandLoop() { while (true) { var settings = new ApplicationSettings("gascommandqueue"); var tokenProvider = new TokenProvider(settings.DeviceAccount, settings.DeviceKey); var rcvClient = new MessagingClient(settings.EventSubmissionUri, tokenProvider); try { var msg = rcvClient.Receive(TimeSpan.FromTicks (TimeSpan.TicksPerSecond * 60), ReceiveMode.ReceiveAndDelete); string command = (string)msg.Properties["command"]; if (command != null) { string commad = (string)msg.Properties["command"]; string owner = (string)msg.Properties["owner"]; string timestamp = (string)msg.Properties["observed"]; if (command == "stop") m_Gas.Stop(); else if (command == "start") m_Gas.Start(); else if (command == "threshold") { double threshold; string t = (string)msg.Properties["param"]; if (double.TryParse(t, out threshold)) m_Gas.SetThreshold(threshold); } Debug.Print("Command received: " + owner + ", command: " + command); } } catch (Exception ex) { Debug.Print("Exception: " + ex.Message); } } } } |
In the next part I will show how to implement the SharePoint 2013 App which directionally communicate with this sensor.
Posted
Dec 09 2014, 08:00 PM
by
Damir Dobric